Since we have a test on Friday, lets do a little bit of a fun activity. One of the badges of honor for the CS major comes from the CS120 class: drawing a fish. This activity was originally created by Dr. N. Jane Ingram and Dr. Adrienne Bloss for their Java labs in the 2000's. We are keeping their tradition alive today.
Details
Create a python program in a file called fish.py. This program should prompt the user to enter two integers, which is the location on the turtle window to draw a line drawing of a fish. Your fish should be drawn centered on the specified coordinate.
Start on paper first!. The activity will progress much easier for you if you figure out the coordinates of various points before you begin coding. Start with a plan and your code will come together very quickly.
Make sure to test your program by running it multiple times with different parameters. Make sure your code follows the course's code conventions.
Example
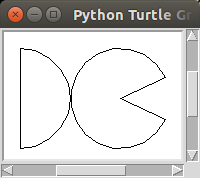
Hint
Challenge
What do you call a fish with no eyes? FSH! Add eyes to your fish so it won't accidentally swim into a dam!
The following exercise summarizes almost everything we have covered so far this semester. Put everything you have learned together in order to format the output of your program correctly.
Details
Create a python program in a file called powers.py. Your program should prompt the user for an integer N, and print (formatted as in the example below) the sum of all of the powers from 1 to N.
Each line of your output should be the equation for computing the sum of the expression: k∑x=1xx=11+22+…+kk You should compute this for all values of k from 1 to N.
Example
$ python3 powers.py How many powers? 10 1 ^ 1 = 1 1 ^ 1 + 2 ^ 2 = 5 1 ^ 1 + 2 ^ 2 + 3 ^ 3 = 32 1 ^ 1 + 2 ^ 2 + 3 ^ 3 + 4 ^ 4 = 288 1 ^ 1 + 2 ^ 2 + 3 ^ 3 + 4 ^ 4 + 5 ^ 5 = 3413 1 ^ 1 + 2 ^ 2 + 3 ^ 3 + 4 ^ 4 + 5 ^ 5 + 6 ^ 6 = 50069 1 ^ 1 + 2 ^ 2 + 3 ^ 3 + 4 ^ 4 + 5 ^ 5 + 6 ^ 6 + 7 ^ 7 = 873612 1 ^ 1 + 2 ^ 2 + 3 ^ 3 + 4 ^ 4 + 5 ^ 5 + 6 ^ 6 + 7 ^ 7 + 8 ^ 8 = 17650828 1 ^ 1 + 2 ^ 2 + 3 ^ 3 + 4 ^ 4 + 5 ^ 5 + 6 ^ 6 + 7 ^ 7 + 8 ^ 8 + 9 ^ 9 = 405071317 1 ^ 1 + 2 ^ 2 + 3 ^ 3 + 4 ^ 4 + 5 ^ 5 + 6 ^ 6 + 7 ^ 7 + 8 ^ 8 + 9 ^ 9 + 10 ^ 10 = 10405071317
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.