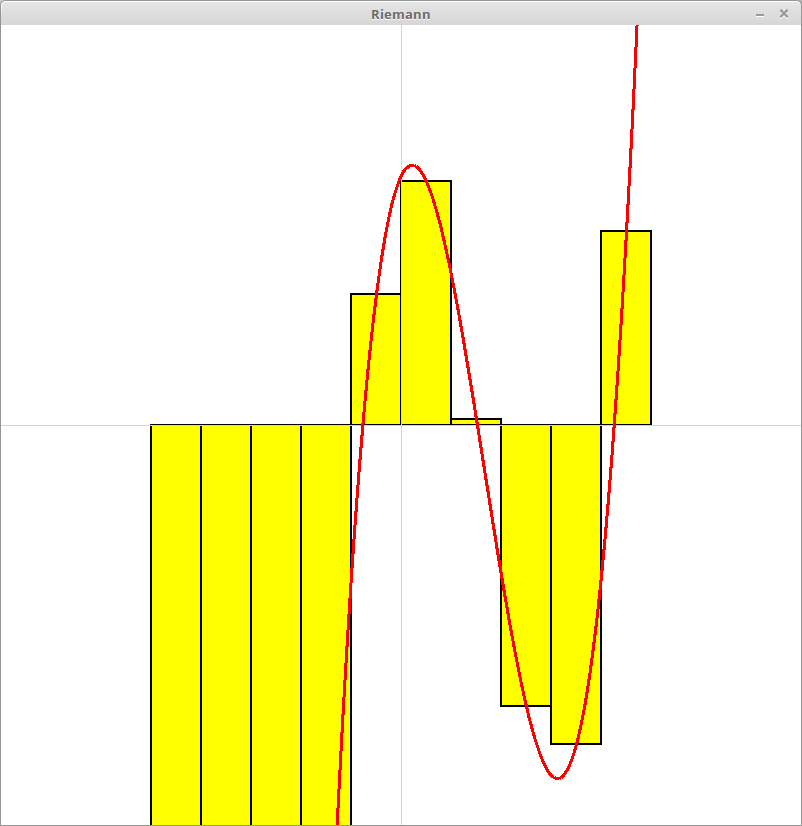
Details
Use the graphics
module, to write a program that can plot an arbitrary polynomial.
To accomplish this, you should define a function
called polynomial(x)
, which takes a floating point
value x, and returns the result of calculating the value of the
polynomial with the value of x. You should define some polynomial
inside of this function. As a starter, use the following
polynomial:
$$f(x) = x ^ 3 - 5 \cdot x ^ 2 + 2 \cdot x + 5$$
Using the defined function above, plot the polynomial on the turtle window. In order to make the plotted function easier to view, you should scale the output of your function up. More on this later.
Once you have plotted the function, you are going to then compute
and plot a series of rectangles to estimate the area under the curve
for some range of values from the domain of the previously defined
polynomial. Define a function called estimate_area(start,
end, size)
, which takes integers for the starting x
value of the range, the ending x value of the range, and
the width of the rectangle used to estimate the area under the
curve.
This function should compute a series of rectangles of the size specified, with a height determined by the value of the polynomial at one of the points specified in that range. Your estimate of area under the curve is just the sum of the area of all of the rectangles combined. Print the estimated value of the area, and draw the rectangles you computed on the Graphics window as well.
Notes
There are two issues we need to deal with, in order to make our plots look realistic. The first is that our origin is in the upper left corner of the graphics window, and that the plots will look really tiny in comparison to the size of the window. We need to scale the drawn shapes to fill a better portion of the window.
Define a global constant called SCALE
. The larger the
value assigned to this variable, the more "zoomed" in on the origin
your drawing will be. We can scale the
function by dividing the input to the polynomial function by this
variable, and multiplying the output of this function by the same value:
Pulling back the curtain a little, this is simply treating each x coordinate as a smaller fraction of the actual x coordinate. The result of the polynomial needs to be scaled up by the same amount, to keep the relative proportions of the function looking correct. This form of scaling will only work in certain scenarios (mostly mathematical functions) but will fit the bill just fine.
The above scaled_output will be the y value associated with the passed in x value. These then need to be shifted so that they are relative to the origin being moved to the center of the screen.
\[ shifted\_x = \frac{width}{2} + x\\ shifted\_y = \frac{height}{2} - y \]These are the points you will use to plot the function, and will be used in positioning of the rectangles as well.
"Hacker" Prompt
Each week, additional exercises related to the assignment will be provided at the end. These exercises are typically more challenging than the regular assignment. Bonus points will be provided to students who complete any of the "Hacker" level assignments.
-
Even Better Estimates: You likely assumed that the value you computed from the polynomial was one of the corners of the rectangle. This provides a decent estimate, but ultimately will always be an over (or under, depending on which corner, etc) estimate of what the value really is. Alter your program so that shifts where you compute the height of the rectangle so that you use the center of the rectangle.
-
Convergence: The area under the curve can be computed by taking the integral of the polynomial. This can be best approximated by computing the area where the size of the rectangles approaches 0. While this is "impossible" for you to currently compute in python, we can compute how much the integral changes as we decrease the width of the rectangles. Use a for loop to compute and print a table of ever decreasing widths of rectangles, and their associated estimate of the area.
-
Square Roots: There are some functions that we can plot, but have some issues that cause Python to not be able to compute values for an area under a curve. For example, \(\sqrt{x}\) cannot be computed, because you cannot compute the square root of negative values, (and the fact that it is not, technically, a function (\(\pm y = \sqrt{x}\))). Create a second program that allows you to plot the square root function.
Grading
The assignment will be graded on the following requirements according to the course’s programming assignment rubric.
Functionality (75%): A functional program will:
-
Have at least two functions,
estimate_area
andpolynomial
-
Plot the
polynomial
to the turtle window. -
Scale the drawing of the
polynomial
function based on the value of some defined constant variable. -
Use Riemann Sums to estimate and print the area under the curve
for some region of the function defined in
polynomial
. - Draw the rectangles used to estimate the area to the turtle window.
Style (25%): A program with good style will:
- include a header comment signifying the authors of the file,
- avoid magic numbers (literal primitive numbers),
- use meaningful names for variables and functions,
- have statements that are small (80 characters or less including leading space) and do one thing, and
- have functions that are small (40 lines or less including comments) and do one thing
- have a comment above functions that includes the purpose, the pre-conditions, and the post-conditions of the function.
- have spaces after commas in argument lists and spaces on both sides of binary operators (=, +, -, *, etc.).
If you've ever created a flip book animation you know that you can create animations by quickly changing images. In this assignment you will create animation like a flip book by moving and changing the image that is displayed.
Details
Create a Python program that uses the graphics module to create an animation of a character running and jumping like the following:
- The program should have a function for the run animation, with parameters that change the start and end locations of the animation.
- The run animation should alternate between two different images so it looks like the character's legs are moving.
- The program should have a function for the jump animation, with parameters that change the start and end locations of the animation.
- The jump animation path should not be linear. It should be circular, parabolic, or sinusoidal to produce a smooth curve.
- The run function should be called twice, before and after the jump. The run function should be called once, in between the run animations. The transitions between the animations should be seamless.
You can use the images below that were used to produce the above animation, but feel free to use any other images you want.
Extra
Create an animation that tells a story. Include text and additional images in the animation.
Grading
The assignment will be graded on the following requirements according to the course’s programming assignment rubric.
Functionality (75%): A functional program will:
- have a run animation function with parameters for the start and end.
- have a jump animation function with parameters for the start and end.
- the run animation should alternate between two images.
- the jump animation should be nonlinear.
- The functions should be called to produce a seamless animation.
Style (25%): A program with good style will:
- include a header comment signifying the authors of the file,
- avoid magic numbers (literal primitive numbers),
- use meaningful names for variables and functions,
- have statements that are small (80 characters or less including leading space) and do one thing, and
- have a comment above functions that includes the purpose, the pre-conditions, and the post-conditions of the function.
- have spaces after commas in argument lists and spaces on both sides of binary operators (=, +, -, *, etc.).
Computers represent colors using red, green, and blue because different combinations of those three lights can be used to produce lots of different colors. But people have an easier time thinking about colors in terms of hue, saturation, and brightness. Fortuneately, it is not difficult to convert between the two representations.
Details
Read about voltage dividers and then create a circuit with an RGB LED and a trimpot. Create a Python program that uses the rotation of the trimpot to change the hue of the LED. The program should have a function that uses an HSL to RGB conversion equations, with constant values for the saturation and lightness, to convert to timpot's rotation to the RGB values. Turning the trimpot through its full range should change the LED though the full range of hues.
Grading
The assignment will be graded on the following requirements according to the course’s programming assignment rubric.
Functionality (75%): A functional program will:
- have a function or functions that convert hue to RGB.
- have a circuit with a trimpot that can be read gPIo.
- have a circuit with a RGB LED that can be conrolled by gPIo.
- control the color of the LED by turning the trimpot.
- normalize the trimpot's range and the LED' to enable displaying the full range of hues.
Style (25%): A program with good style will:
- include a header comment signifying the authors of the file,
- avoid magic numbers (literal primitive numbers),
- use meaningful names for variables and functions,
- have statements that are small (80 characters or less including leading space) and do one thing, and
- have spaces after commas in argument lists and spaces on both sides of binary operators (=, +, -, *, etc.).
Submission
Submit your program as a .py file on the course Inquire page before class on Wednesday October 5th.