This assignment was inspired by XKCD What if? #24: Model Rockets
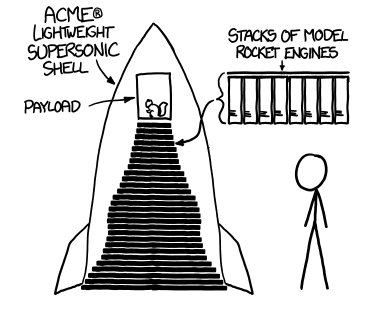
Over the past 50 years, spaceflight has become more technologically advanced. While NASA's prospects on manned spaceflight have fizzled out, commercial spaceflight is just around the corner. For the low, low price of $250,000, you to could travel to the edge of space and back. However, one does not necessarily have to wait that long to get to space, or spend that much money...or do they?
Estes is a company that makes model rocket engines. One of their most powerful, but commonly available, model engines is the E9. The E9 engine provides 28.5 Newton/second of thrust. Given that an average human being weighs about 160 pounds, let's figure out how many model rocket engines are needed to lift the human off the ground.
Details
Create a program called lift_off.py
. This program should
print the information about lifting a human off the ground. In
particular, your program should print:
- The equation (as text) you derived to determine the number of rocket engines required to lift the human off the ground,
- How many rocket engines are required to lift a 160 pound human being, Assuming all thrust is additive,
- Assuming three rockets come in a pack, how many full packs of rockets are used,
- In the (potentially) not fully used pack, how many rockets are left over,
- And assuming each rocket engine weighs 35.8 grams, how much the collection of rocket engines weighs.
Remember 1 N = 1 kg/s. For a vertical launcing rocket, lift-off requires the thrust to exceed the the gravitational force (9.81 N) on the object being lifted. You can assume weightless rockets for the computation of the forces, though this is generally a bad assumption.
"Hacker" Prompt
Each week, additional exercises related to the assignment will be provided at the end. These exercises are typically more challenging than the regular assignment. Bonus points will be provided to students who complete any of the "Hacker" level assignments.
- Cost of travel: For this mechanism to be worth while, we really need to know how much it would cost to lift an average human off the ground. Assuming each pack of rockets costs $54, how much does the launched monstrosity cost?
- To Infinity: Of course, that's just to get the person off the ground. Where's the fun in that?!? Compute how many rockets would be necessary to get the human 1000m in the air! You can assume all thrust is immediate.
- What if?: The idea for this assignment came from the web site xkcd what if? Read through some other questions, and create a program that performs the calculations.
Grading
The assignment will be graded on the following requirements according to the course’s programming assignment rubric.
Functionality (75%): A functional program will:
- print the derived equation,
- print how many rocket engines are required to lift a 160 pound human being,
- print how many full packs of rockets are used,
- print how many rockets are in the leftover pack, and
- print how much the collection of rockets weights
Style (25%): A program with good style will:
- include a header comment signifying the authors of the file,
- avoid magic numbers (literal primitive numbers),
- use meaningful names for variables,
- have statements that are small (80 characters or less including leading space) and do one thing, and
- have spaces on both sides of binary operators (=, +, -, *, etc.).
Computers can be powerful tools for artists. Their ability to represent curves and lines precisely make them a useful tool for graphic designers. In this assignment you will use the Turtle module as a computer aided design tool.
Details
Create a Python program that uses the turtle module to draw a yin-yang symbol like the following:
- The radius of the drawing should be specified with a variable. Changing this variable should change the size of the entire drawing without changing the drawing in any other way.
- The drawing should be centered in the window.
- The small inner circles should be 1 / 6 of the size of the outer circle.
- Use the circle, begin_fill, and end_fill functions to draw filled shapes.
Extra
Add anything you want or create an entirely new drawing. Be creative and impress me.
Grading
The assignment will be graded on the following requirements according to the course’s programming assignment rubric.
Functionality (75%): A functional program will:
- use a variable for the radius of the drawing.
- draw the picture centered in the window.
- use
begin_fill
andend_fill
to color the drawing correctly. - draw two interlocking, curved, teardrop-shapes with the correct size and location.
- draw two small circles that are the correct size and location.
Style (25%): A program with good style will:
- include a header comment signifying the authors of the file,
- avoid magic numbers (literal primitive numbers),
- use meaningful names for variables,
- have statements that are small (80 characters or less including leading space) and do one thing, and
- have spaces on both sides of binary operators (=, +, -, *, etc.).
Details
Read about light-emitting diodes and then create a circut with a single LED and Python for the Raspberry Pi that will make the LED blink SOS, that is short-short-short-long-long-long-short-short-short. The program should use variables for the length of the short flashes, long flashes, and time between flashes.
Before creating the above circuit and program, you will need to set up and learn how to use the Rasberry Pi. Do the following tutorials:
- Raspberry Pi 2 Starter Kit Hookup Guide (you can skip WiringPi section)
- Rasberry gPIo Tutorial (you can skip the WiringPi sections)
Grading
The assignment will be graded on the following requirements according to the course’s programming assignment rubric.
Functionality (75%): A functional circuit and program will:
- have setup the Rasberry Pi according to the hookup guide.
- have a cicuit set up according to the Raspberry gPIo tutorial.
- have variables for the blink durations and time between blinks.
- blinks the LEDs in a SOS pattern.
Style (25%): A program with good style will:
- include a header comment signifying the authors of the file,
- avoid magic numbers (literal primitive numbers),
- use meaningful names for variables,
- have statements that are small (80 characters or less including leading space) and do one thing, and
- have spaces on both sides of binary operators (=, +, -, *, etc.).
Submission
Submit your program as a .py file on the course Inquire page before class on Wednesday September 14th.