All computer memory is binary, so whether an integer is represented as a string or as a number, it is still represented as a number in binary.
Details
Write a function called parse_binary(binary_string)
in
a file called parse_bin.py. The function should return an
int value that is equivalent to the binary integer represented
with binary_string
. The function should assume
that binary_string
consists of only the characters '0'
and '1'.
Make sure your program handles all necessary cases gracefully. What additional test cases should you check?
Sample Test Cases
Function Call | Expected Output |
---|---|
parse_int('101') | 5 |
type(parse_int('101')) | <class 'int'> |
Hint
Challenge
int_to_binary_string(an_int)
, which takes an
integer as input. Your function should return a string of 1's and
0's, the binary representation of the input integer.
Cryptography can be used to make the contents of a message secret, but sometimes you want to hide that a message is even being sent. Steganography is one way to hide messages, and a common technique takes advantage of our inability to perceive small changes in colors. For example the image below consists of two boxes with different colors.
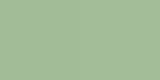
The left box has the RGB values (161, 187, 150). The box on the right has the RGB values (162, 188, 151). The two boxes appear to be the same color because the RGB values are similar. A message can be hidden in an image by making small changes to the RGB values of an existing image.
A common way of modifying an image is to change only the least significant bits of the RGB values. The least significant bit of a number is the right-most bit and represents the value 20, or 1. Therefore, the most a color value will change if the least significant bit is change is 1, which the above image demonstrates as imperceptible. The following whale image, for example, has a message encoded in it.
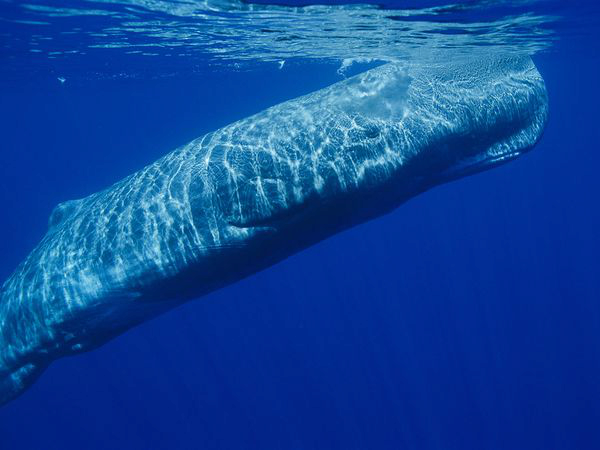
Details
Create a function called decode_image(ppm_file_name)
in
a file called steganography.py
. The program should
return the secret message that is embedded in the specified image
file. The message is encoded as 8-bit ASCII in the least
significant bit of the image's color data. Assume that the number
of color values in the file is a factor of 8. Once you have tested
your program on a simple example, you can test it on the
above
whale image.
Some things to keep in mind:
-
The first three lines of a PPM file specify header information for the image. You will have to skip these three lines.
-
Each subsequent line specifies one color value of a pixel (Red, Green, and Blue respectively). You need to extract the least significant bit of each of these values.
-
Once you have processed 8 color values, you will have to convert the binary sequence represented by their least significant bits into a single character. This is one character in the hidden message.
Example
>>> image_file = open("demo.ppm", "r") >>> print(image_file.read()) P3 1 8 255 122 101 215 42 2 128 200 13 64 203 155 130 36 172 91 180 88 77 55 22 44 66 33 99 >>> print(decode_image("demo.ppm")) abc
Hint
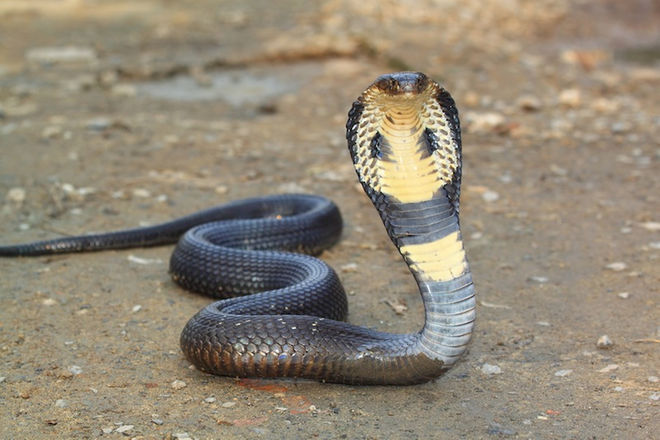
Challenge
Write a function that can embed messages into images. The function should take three parameters: a message, an input image file name, and an output image file name. It should read in the input image, modify the least significant bits of the color values to embed the message, and write the new image to the specified output file.
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.