Practice Problem 1
Write a function double_all(a_list)
, which takes
a
list of floating point numbers. Your function should
return a
version of a_list with all values doubled.
Practice Problem 2
Write a function remove_all(a_list, an_element)
,
which
takes a list and some element. Your function should remove
all
occurances of an_element from a_list, and return the
resulting list.
Flappy Bird was an incredibly popular game back in the Spring of 2013. It made A LOT of money, especially considering it was a "free" game. The game was suddenly removed from the App Store, but the initial success of the game resulted in many clones being available almost instantly. The overall game is not super difficult to replicate, now that we have lists!
Details
Create a python program in a file called flappy.py. This file will replicate a somewhat simplified version of the Flappy Bird game. Towards this end, you will want to replicate the gravity function demonstrated in class earlier today. Don't forget to place the graphics module and sprite module in your directory.
Every 3 seconds, a new pipe should be generated. It should start at the right side of the screen, and slowly work its way to the left side of the screen. The height of the pipe should be randomly generated. Every pipe generated should be stored in a list, so that all of the pipes can be updated at once.
Create a function called move_pipes(pipe_list)
,
which takes a list of Sprite objects which represent pipes in the
game. This function should move all of the pipes to the left by the
same amount. If a pipe is past the left edge of the screen, you
should remove.
Also create a function called check_collision(bird_sprite,
pipe_list)
, which takes the bird sprite object and a list of
the pipe objects. It should return True if the bird sprite
is colliding with any of the pipes.
Use these two functions to replicate a simple version of flappy bird, with pipes only on one side (bottom) of the screen.

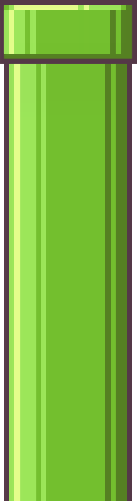
Example
Hint
-
Every sprite object has 2 important attributes: x
and y. These are the x and y coordinates the sprite
is being drawn at. You can update these directly, and those
values will be used the next time you call
graphics.draw_sprite
. - The sprite is drawn centered at the specified x and y coordinates. When you generate the new y coordinate for the sprite, you should compute the y coordinate for the top of the pipe. Then add half the height of the pipe of the pipe to get the center y coordinate of the pipe.
- It might seem natural to delete items from the list as you are looping over its elements. However, modifying that list will likely result in unforseen runtime errors. Because of the structure of the list, only the first element has a chance of being off the screen. Instead of checking each element, you only need to check the sprite at index 0.
Challenge
Modify your program so that there are pipe sprites along the top of the screen as well. You will either need to flip the pipe sprite, or find a different sprite for the top.
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.