In Ubuntu on the lab machines, search for, and run, “Hello Code”.
Complete start_level(0)
Challenge
Complete start_challenge(0)
In Emacs, create a Python program in a file called age_in_seconds.py. In this file, we will compute and output a rough estimate of your age in seconds.
Create the following variables in your program, with the appropriate values for each:
- DAYS_PER_YEAR
- HOURS_PER_DAY
- MINUTES_PER_HOUR
- SECONDS_PER_MINUTE
Note these are all constants, so they are written in all upper case.
Using these variables, we can compute the value of an
additional SECONDS_PER_YEAR
variable by multiplying all
of the above together.
Create a age_in_years
variable, and use it to store
your current age in years. Use this variable, as well as
the SECONDS_PER_YEAR
variable to compute the value for
a final variable age_in_seconds
.
Don't forget to print the value
of age_in_seconds
, in a format similar to below!
Example
$ python3 age_in_seconds.py Scotty's age in seconds is 977616000
While you might not be aware of it, Roanoke College switched their MaroonCards to a completely new system. This sounded like a great idea to us, so we figured we could create some ID cards using Turtle for the entire class!
Details
In Emacs, create a Python program in a file called ID_card.py in your lab2 directory. In this program, you are going to use turtle to print the name and intended major of both yourself and your lab partner. Your name should appear on the left side of the screen, and your major should appear directly below it. Your partner's name should appear on the right side of the screen, and their major should appear right below their name.
You
turtle.window_height()
and
turtle.window_width()
commands to retrieve the width and height of the Turtle window, and
use these commands to compute the y-location of the names and majors.
Make sure you use variables where appropriate. You may also draw any additional patterns as you wish on your card. Just make sure the text is still legible!
Example
Note: The text in the following image has been enlarged to improve readability. Your text does not need to be this large
$ python3 ID_card.py
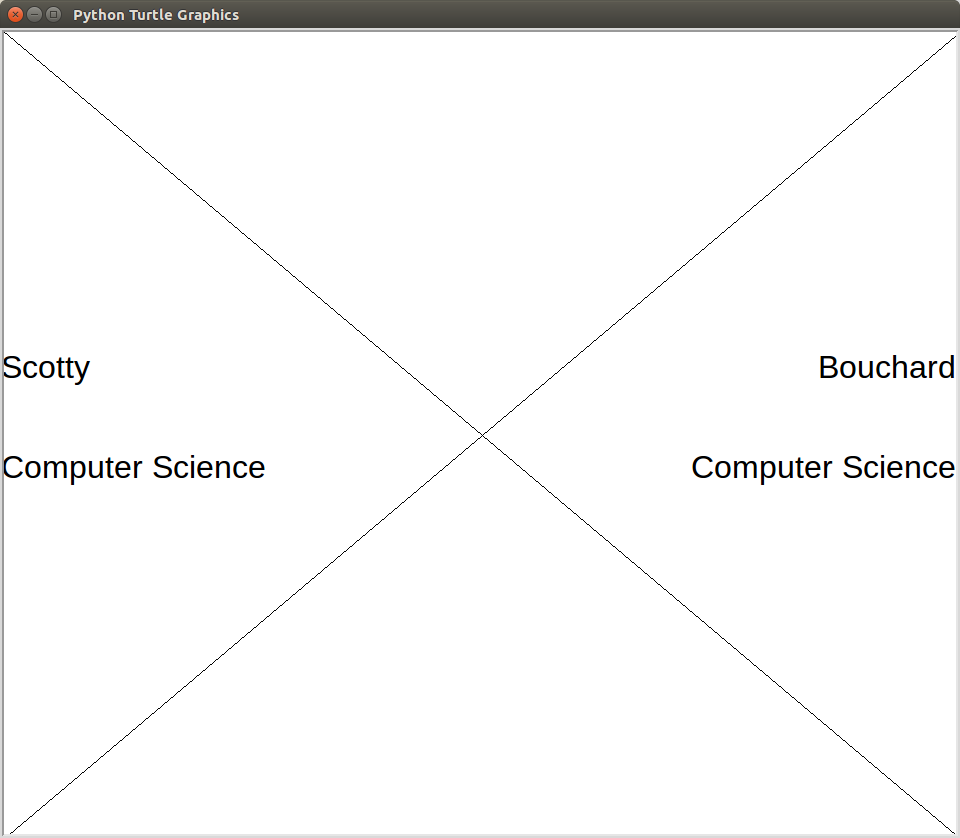
Hint
-
The Turtle Window's origin is the center of the screen. To get the x and y coordinates of the edges of the screen, you will have to divide the width and height of the screen by 2.
-
Turtle will, by default, write all text to the left of the current location of the Turtle. You will need to modify the starting position of the turtle to place it along the right edge.
-
You can move the Turtle using the standard
forward
,left
, andright
commands with little trouble. However, it might be easier to usegoto
. Either way, you probably want to make sure the turtle's pen is up!
Challenge
This process could even be created for a roster of students, as opposed to ID cards. You probably don't have enough time to talk to everyone in class, but talk to at least two other people, and add their name and intended major to the screen. One to appear on the top edge of the screen, and the other to appear along the bottom edge.
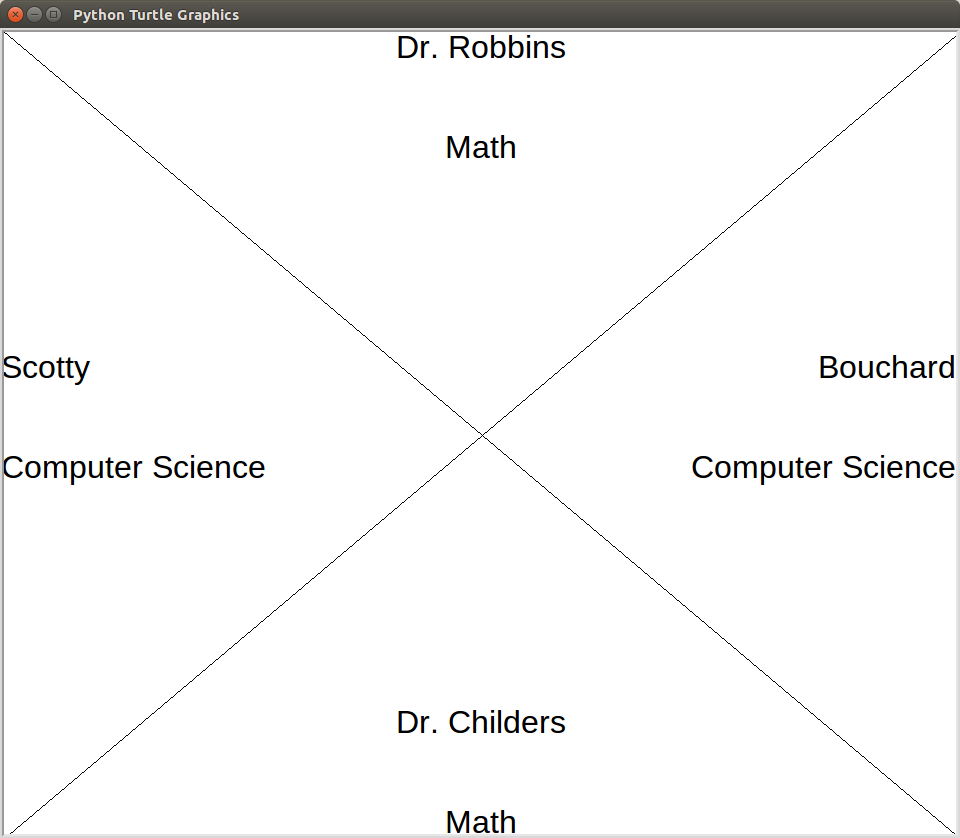
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.