Create a python program which generates 10000 random numbers using
the random.random()
function. Compute the sum of these
values, and print the average of the generated numbers.
Example
$ python3 practice1.py The average random number is 0.4999748999511569
Write a python program which prompts the user for values \(a, b, \mbox{ and } c\), and computes the positive solution to the quadratic formula:
\[ x = \frac{-b + \sqrt{b^2 - 4 \cdot a \cdot c}}{2 \cdot a} \]Example
$ python3 practice2.py a? 2 b? 3 c? 1 x = -0.5
We have already seen how we can use an accumulator to compute \(n!\) for an arbitrary value of \(n\). The problem we run into, however, is that our loop can take a long time to run for large values of \(n\). This means that having a closed form approximation might be desirable instead. Luckily, several approximations have been developed, most notably by Stirling and Gosper.
Details
In Emacs, create a Python program in a file called fact_approximation.py that prompts the user to input a value \(n\), and prints the values of \(n!\), \(\ln{n!}\), and the approximations of \(\ln{n!}\) and \(n!\) using Stirling's and Gospers' approximations.
Gospers' approximation is defined as follows:
\[ n! \approx \left(\frac{n}{e}\right)^n \cdot \sqrt{\left(2 \cdot n + \frac{1}{3}\right) \cdot \pi}\ \]Where \(e\) is Euler's Number (and is provided by the Math module). Stirling's approximation is defined as follows:
\[ \ln{n!} \approx n \cdot \ln{n} - n + \frac{1}{2} \cdot \ln{\left(2 \cdot \pi \cdot n\right)} \]Where ln is the natural log (again computed using the math module).
Example
$ python3 fact_approximation.py n: 5 5 ! = 120 Gospers' Approximation: 119.97003016968553 ln( 5 !) = 4.787491742782046 Stirling's Approximation: 4.7708470515922246
Hint
There are 4 components of the math library that you need to
use in this program: math.pi
gives you the
python approximation of π, math.e
which
gives you the python approximation of \(e\),
math.factorial
which is a function you can use to
compute the factorial of a number, and math.log
which can be used to compute logarithms. You can also
use math.sqrt
to compute the square root of a
number as well.
Challenge
Gosper's approximation works well for the first 7 integers, but quickly starts to lag behind the true values. Stirling's approximation stays relatively close to the requested values. Modify your program to compute the average error (the difference between the approximations and the real values) for the first 100 integers. Print these values at the end of your program.
Back before modern flat LCD displays, computers used Cathode Ray Tube, CRT, displays. Besides being big and heavy, the displays suffered from burn-in. If the same image was left on the display too long, a ghost of it would become permanent. So, if a computer was not used for a short duration, it would play an animation to save the screen from burn-in. We don't need screen savers anymore, but computer still come with them because they are fun to look at.
Details
Create a python program in a file called screen_saver.py. The program should use the graphics module to draw lots of ovals of random sizes in random locations. The ovals can be any size, but random locations must be limited to and fill the dimensions of the window.
Example
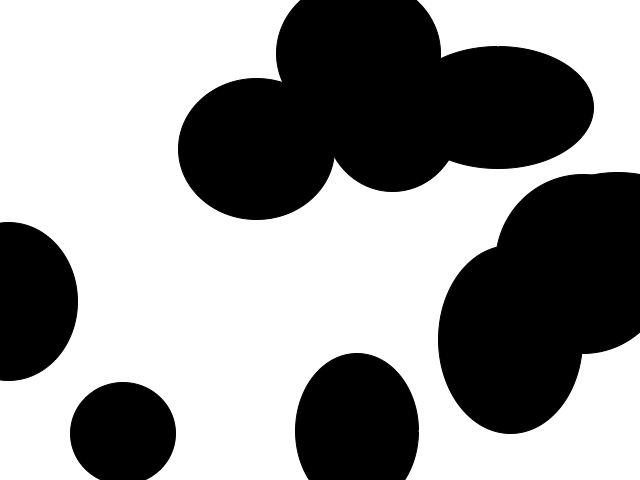
Hint
-
Use a for loop and the graphics
module's
draw_oval
function to draw many ovals. -
Use the random module's
randrange
function to generate a random x-coordinate, y-coordinate, width, and height for each oval. -
Use the graphics modules
window_width
andwindow_height
functions to limit the range of the x and y coordinates.
Challenge
After awhile the screen is all black, not a very interesting animation. In order for the amimation to continue longer, it should have lots of colors. Make each oval drawn a random color.
Example
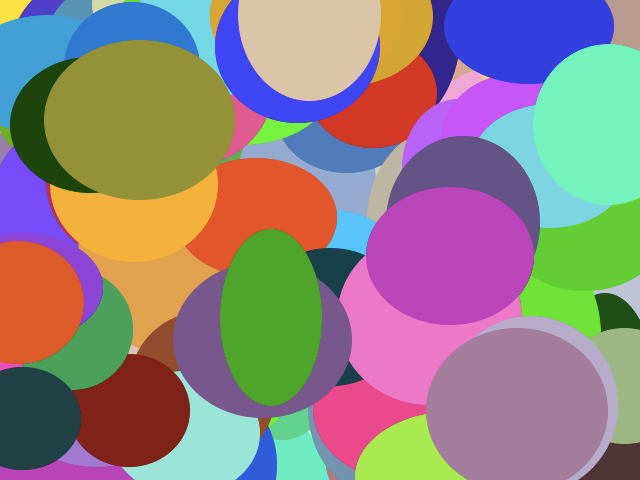
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.