Write a Python program that uses turtle graphics to draw a diamond.
Example
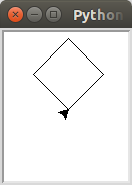
Write a Python program that uses turtle graphics to draw a square that is centered in the window.
Example
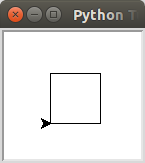
One of the first shapes you learned to draw in grade school was probably a star. It is a pretty easy shape to draw, looks pretty cool, and can be done entirely with straight lines. The only tricky part is determining how to go from one point of the star to the next. Today, you will figure that part out.
Details
In Emacs, create a Python program in a file called star.py. Your program should use the turtle module to draw a five-pointed star.
Example
$ python3 star.py
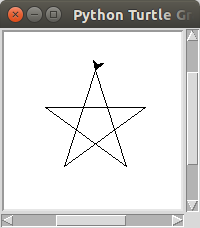
Hint
- Drawing the star is much easier if you use the
functions
turtle.forward
and eitherturtle.left
orturtle.right
. - Note that the turtle will need to turn once for each point of the star and that it will turn a total of 720°. So, you can calculate the number of degrees in each turn.
- Finally, drawing the star is simply alternating between moving forward and turning.
One of the great advantages to using a computer for drawing is that it is possible to draw precisely. It makes creating something perfectly symmetrical very easy.
Details
In Emacs, create a Python program in a file called house.py. Your program should use the turtle module to draw a simple house. The house should be a square with a triangle for a roof, and a rectangle for a door. The house should be symmetrical about a vertical line.
Example
$ python3 house.py
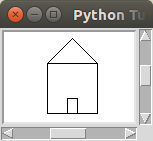
Hint
Challenge
Spruce up the house. Add things like a chimney, the sun, a tree, a mailbox, a fence, whatever you want, as long as it impresses me.
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.