Min 2
Write a function called min_2(number_1, number_2)
that
returns the minimum of the two parameters, number_1
and number_2
. The function should not use the
built-in min
function.
Test Cases
print('Input: 0, 0\t\tActual:', min_2(0, 0), '\tExpected: 0') print('Input: 1, 2\t\tActual:', min_2(1, 2), '\tExpected: 1') print('Input: 1, -2\t\tActual:', min_2(1, -2), '\tExpected: -2') print('Input: 1.5, -2.5\tActual:', min_2(1.5, -2.5), '\tExpected: -2.5')
Min 3
Write a function called min_3(number_1, number_2,
number_3)
that returns the minimum of the 3 parameters to
the function, number_1
, number_2
,
number_3
. The
function should not use the built-in min
function.
Test Cases
print('Input: 0, 1, 2\tActual:', min_3(0, 1, 2), '\tExpected: 0') print('Input: 0, 2, 1\tActual:', min_3(0, 2, 1), '\tExpected: 0') print('Input: 1, 0, 2\tActual:', min_3(1, 0, 2), '\tExpected: 0') print('Input: 2, 0, 1\tActual:', min_3(2, 0, 1), '\tExpected: 0') print('Input: 1, 2, 0\tActual:', min_3(1, 2, 0), '\tExpected: 0') print('Input: 2, 1, 0\tActual:', min_3(2, 1, 0), '\tExpected: 0')
Point in Circle
Earlier this semester we estimated π using the Leibniz approximation. Another way of estimating the value of pi is to use Monte Carlo Estimation. The technique is named after the casino because it depends on random chance and probability. A program that estimates π this way must test whether a point is inside of a circle.
Details
Create a Python program that uses the graphics module to allow the user to draw dots. The program should draw a large circle centered in the window. When the user clicks on the window, the program should draw a dot as a small circled centered around where the user clicked. The color of the dot should be determined by whether or not the dot is inside the large circle. If the center of a dot is inside the circle, the dot should be green, if the center of a dot is outside the circle, the dot should be red. Assume a dot exactly on the edge of the large circle is inside the circle.
Example
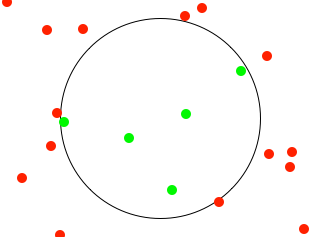
Hint
-
A point is inside of a circle if the distance between the point and the center of the circle is less than the radius of the circle.
The distance between two points can be computed using the Euclidean Distance formula:
d=√(x1−x2)2+(y1−y2)2
Where d is the distance between the points (x1,y1) and (x2,y2).
-
The program can use
graphics.wait_for_button_press()
to suspend execution of the program until the user clicks a mouse button. -
The program can use the
x
andy
functions to get the coordinates of the mouse click.
Challenge
To perform a Monte Carlo estimation of π, we need to estimate the area of the circle. Create a program that draws a large circle circumscribed by a square in the center of the window. That is, the width and height of the square is the same as the diameter of the circle. The program should generate a large number of random points. It should be possible for a random point to be located anywhere inside the square but nowhere outside of the square. The fraction of points that are inside the circle is an estimate of the area of the circle as a fraction of the area of the square. Since the area of a circle is πr2, the program can use the estimated area to solve for π
Bouncing
By drawing inside of loops we can create animations. With the addition of conditional statements we can create less prescribed and more dynamic animations. One such animation, which you have likely seen on every DVD player since the 1990's, is a logo which continually bounces around the screen. Today, using the graphics module and conditional statements, you will replicate a very simple form of this screen saver.
Details
Create a Python program that animates an image bouncing back and forth in the window. The image should begin by moving to the right edge of the screen. Once it reaches the right edge of the screen, the image should "bounce" and move to the left edge of the screen. Note: the program should have only one loop, but the image should bounce multiple times.
Example
Hint
-
The program should have a for loop where each
iteration the image is drawn at a different location. Do not
forget the
graphics.clear
andgraphics.wait
functions. - Create variables to represent the image's x and y coordinates. The variables should be defined before the animation loop.
- In addition to position, you need to store a variable for the images's speed.
- Use the accumulator pattern to update the x location of the image by the image's speed.
- If the image 'hits' the edge of the graphics window, change the direction of the image by changining its speed.
- Use a conditional statement comparing the image's x coordinate to the width of the window to determine if the image has 'hit' the edge of the screen.
Challenge
To make a better screen saver, the image should move diagonally so that it can hit the top and bottom edges of the window as well.
It also looks better if the image never leaves the window. Modify the conditional statements so that it looks like the image bounces as soon as it hits the edge of the window.