Create the function draw_triangle(pen)
that draws a
triangle. The parameter pen
is a turtle object to use
to draw the triangle. The triangle can be any orientation and can
be any size.
Example
gertrude = turtle.Turtle() draw_triangle(gertrude) gertrude.up() gertrude.forward(50) gertrude.down() draw_triangle(gertrude)
Create the function draw_circle(pen, x_loc, y_loc,
radius)
that draws a circle. The parameter pen
is a turtle object which you can use to draw the circle. Note that
the turtle may be located anywhere and have any heading. The
parameters x_loc
and y_loc
are the center
of the circle. The parameter radius
is the radius of
the circle.
Example Output
gertrude = turtle.Turtle() num_circles = 3 size = 50 y = 0 for i in range(num_circles): x = size * i draw_circle(gertrude, x, y, size)
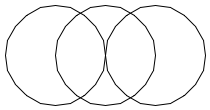
Fun Output
num_circles = 100 size = 100 RADIANS_IN_CIRCLE = 2.0 * math.pi for i in range(num_circles + 1): x = size * math.cos((i / num_circles) * RADIANS_IN_CIRCLE) y = size * math.sin((i / num_circles)* RADIANS_IN_CIRCLE) - (size * 2.0) draw_circle(gertrude, x, y, size)
Another benefit of functions is to save on repeated code. There are some things we do A LOT in our programs, and many things that we do across many programs. One of these is drawing a filled square, which you will implement today.
Details
Download and open in Emacs the Python program
called pixel_art.py. In
this file, there is an empty function definition
fill_square(pen, color, x_loc, y_loc, size)
. This
function should accept a turtle object, a string representing a
turtle color, integers x_loc and y_loc representing a location on
the turtle window, and an integer > 0 which is the size of one of
the sides of the square. Your function should draw a filled square
of the correct dimensions centered at the specified
(x_loc, y_loc).
Run your program and make sure you get the correct output. Also, make sure your code follows the courses code conventions.
Example
$ python3 pixel_art.py
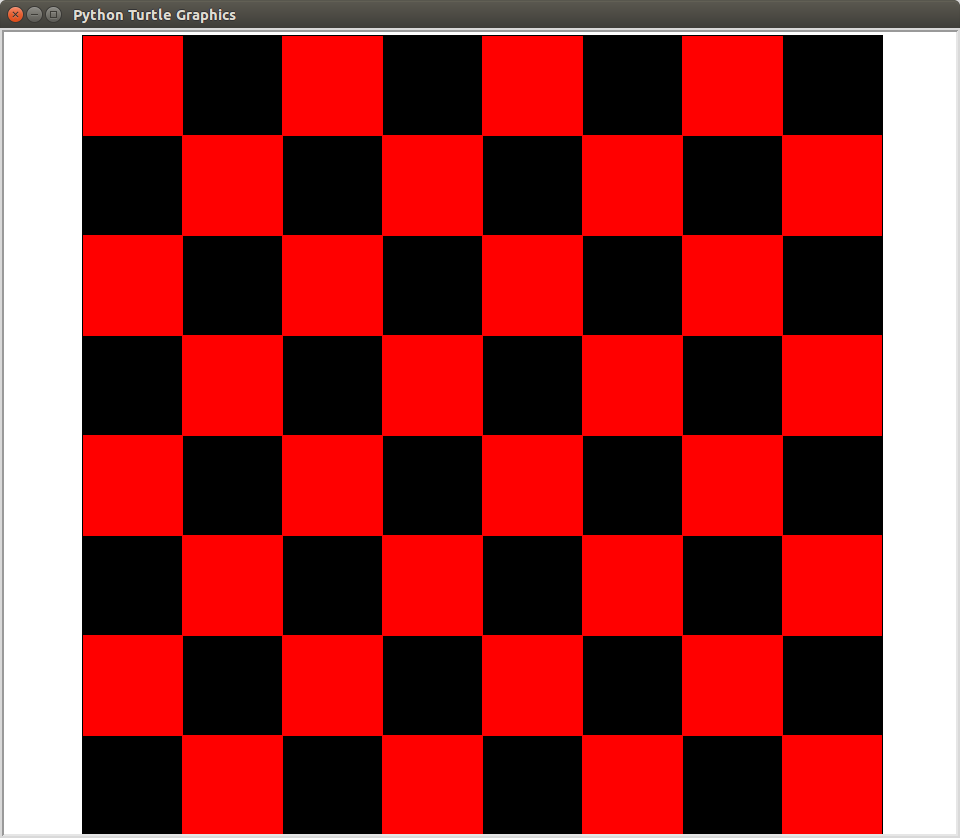
Hint
- The parameters for this function include a turtle object called pen. Using this "variable" within your function will allow you to control whichever turtle was given to you. Use pen for ALL turtle movements.
- The (x_loc, y_loc) parameters to the function specify the center of the square. You need to shift the position of the pen by half of the size in both the x and y directions to center the square drawn.
- Drawing the square is pretty easy, you just need to go forward and turn right 4 total times.
-
Make sure you use
the
color
,begin_fill
andend_fill
functions for setting the turtles color, as well as causing the shape to get filled in with color afterwards.
Challenge
This function allows you to draw an arbitrary filled in block at some location on the turtle window. "Old-school" computer graphics relied on this type of art to create visible shapes on the screen. This is known as pixel art, and there are TONS of examples around the Internet. Either copy your favorite, or create your own!
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.