Use the command line to create a new directory called lab7 in your labs directory. Make sure all of the .py files that you create for this activity are in that directory.
A convex regular polygon is a shape that consists of a sequence of straight lines that all have the same length and meet at the same angle. Convex regular polygons can be parameterized according to the number of sides the shape has. For example, the convex regular polygons with 3, 4, and 5 sides are an equilateral triangle, a square, and a pentagon. As the number of sides increases the names of the polygons get more complicated, for example a 36-sided convex regular polygon is called a triacontakaihexagon. In this activity you will create a function that can create any convex regular polygon.
Details
Create the function draw_polygon(num_sides,
circumference)
in a file called polygon.py. The
function should use the turtle module to draw a convex
regular polygon. The num_sides
and circumference
parameters specify the number of
sides and the circumference of the polygon to draw.
Test your function by calling the function multiple times with different parameters. Make sure your code follows the courses code conventions.
Example
turtle.up() turtle.goto(-100, 0) turtle.down() draw_polygon(3, 200) turtle.up() turtle.goto(0, 0) turtle.down() draw_polygon(4, 200) turtle.up() turtle.goto(100, 0) turtle.down() draw_polygon(5, 200)
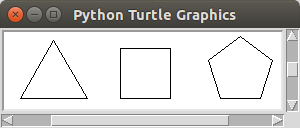
Hint
- Drawing a convex regular polygon is a lot like drawing a
star, it is easier if you use the
functions
turtle.forward
and eitherturtle.left
orturtle.right
. - Unlike the star, you will need to use a for loop to draw the
polygon. Because the function must work for any number of
sides, the number of times the turtle moves forward and turns
will be different each time the function is called. Use the
num_sides
parameter to control the number of times the loop runs. - Also just like drawing the star, the turtle will need to turn once for each corner of the polygon of the star. So you can calculate how much it should turn because the sum of all of the turns will total 360°.
- Calculate the distance the turtle moves forward from the circumference of the polygon. The turtle will move forward the same distance every time, so the sum of every forward movement must be the circumference of the polygon.
Challenge
As the number of sides in a convex regular polygon approaches infinity, it becomes a circle. Write a function that takes advantage of this to draw a circle. The function should have one parameter for the radius of the circle. Note that at some point adding more edges to a polygon approximating a circle does not produce a better looking circle, but the number of edges depends on the size of the circle. The function should also calculate the number of edges needed to draw a good looking circle based on the radius of the circle.
Just like the flip book animations you created in grade school, creating an animation on a computer is simply displaying a sequence of slightly different images. Because this consists of doing the same thing repeatedly, drawing an image, it is a perfect application of loops.
Details
Create a function called animate_image(image_name)
in a
file called animate.py. The function should use
the graphics module to animate an image
moving from the left side of the window to right side of the window
and back. The image_name
parameter specifies the name
of the image file to animate. You can download and use any image
file you want, but the image must be in the same directory as
the animate.py file and it must be a gif file. The image
should traverse the full extent of the window, but should not go
outside the bounds of the window.
Test your function by calling the function multiple times with different parameters. Make sure your code follows the courses code conventions.
Example
for i in range(4): animate_image('red_oval.gif')
Hint
To animate the image, it must be repeatedly erased and drawn. Use a for loop that for each iteration should:
- clear the window
- draw the image at a slightly different location than the previous iteration.
Use the for loop's iterator variable to specify where the image is drawn.
By default, the window size is 640 x 480 pixels. You can use
this information, as well as the information about the size of
the image you are drawing to determine the necessary parameters
to the range
function.
Challenge
Create a new function that can animate two different images moving in two different directions simultaneously.
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.