Use the command line to create a new directory called lab6 in your labs directory. Make sure all of the .py files that you create for this activity are in that directory.
Functions are great for code reuse. A well written function can be used in many different programs.
Details
In Emacs, create a Python program in a file called rectangle.py. Your program should use the turtle module to draw several rectangles on the turtle window.
Your program should define the function draw_rectangle(x, y,
width, height)
. The parameters x and y specify
the location to draw the rectangle on the screen. The width
parameter specifies the size of the rectangle in the x direction,
and the height parameter specifies the size of the
rectangle in the y direction.
Example
>>> rectangle.draw_rectangle(x=0, y=0, width=10, height=25) >>> rectangle.draw_rectangle(x=10, y=15, width=15, height=5)
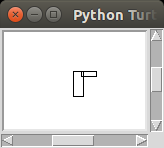
Hint
Challenge
Write a function to draw an octagon. The function should have parameters to specify the location and size of the octagon to draw. Use it to draw several octagons on the turtle screen.
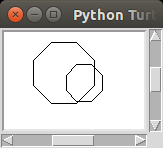
A benefit of writing functions is that we can use them to simplify complex programs. For example, you now have a function written to draw a square. You can use that function to create another function that draws something with multiple squares. And you can use that function to create even more complex drawings.
Details
In Emacs, create a Python program in a file called house.py. Your program should use the turtle module to draw a house in the turtle window.
You need at least two new functions: draw_triangle(x,
y, side_length)
and draw_house(x, y)
. Both of
these functions should have parameters to make drawing with these
functions easier.
Example
>>> house.draw_house(-100, 0) >>> house.draw_house(100, 0)
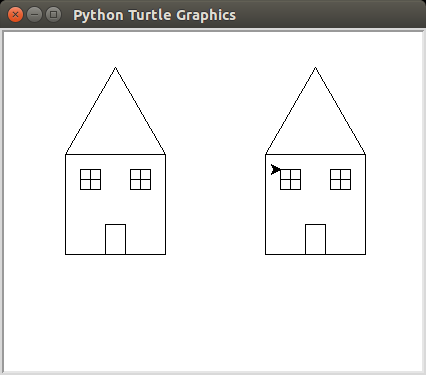
Hint
Challenge
Your drawing probably looks pretty bland as of right now. However, you can easily add color to your drawings using the turtle module. Add color to at least the door and roof of the house, and add a green rectangle to the scene to represent grass.
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.