Use the command line to create a new directory called lab5 in your labs directory. Make sure all of the .py files that you create for this activity are in that directory.
One of the first shapes you learned to draw in grade school was probably a star. It is a pretty easy shape to draw, looks pretty cool, and can be done entirely with straight lines. And on the plus side, you can show off your patriotism by drawing several of them at once. To get started, let's try to draw just a single star.
Details
In Emacs, create a Python program in a file called star.py. Your program should use the turtle module to draw a five-pointed star.
Your program should have a variable for the length of the lines that make up the star. It should also use arithmetic expressions that calculate angles needed to draw the star. Make sure you use proper variable names follow the course's code conventions.
Example
$ python3 star.py
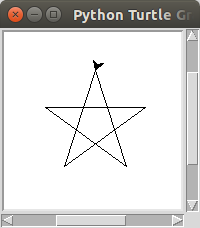
Hint
Computer displays are made up of a grid of tiny lights. Because the displays are not continuous, curved objects must be broken into many non-continuous pieces. So curves are often simply represented as many straight lines. In this activity you will approximate a curve by drawing many lines.
Details
In Emacs, create a Python program in a file called curve.py. Your program should use the turtle module to draw a curve using overlapping straight lines between the x-axis and the y-axis.
Do not hard code the location of the line segment end points. Your program should have a variable that determines the size of the drawing. Your program should calculate the end point locations based on the size variable. Make sure you use proper variable names and follow the courses code conventions.
Example
$ python3 curve.py
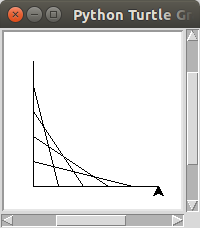
Hint
Challenge
This same technique can be used to draw a myriad of different curves, by changing the length and angle of the axes. Add variable to your program that specify the length and angle of each of the axes to create curves like the following.
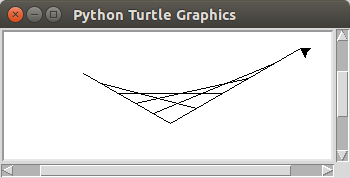
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.