Use the command line to create a new directory called lab31 in your labs directory. Make sure all of the .py files that you create for this activity are in that directory.
In Conway's game of life, a two dimensional grid represents a petridish. Each cell in the grid is either occupied by a bacteria (1), or not (0). In each turn of the game every cell in the grid changes state based on the following rules:
- If a cell is occupied and fewer than 2 of the 8 adjacent cells are occupied, the bacteria dies due to loneliness and the cell becomes unoccupied.
- If a cell is occupied and more than 3 of the 8 adjacent cells are occupied, then the bacteria dies due to overcrowding and the cell becomes unoccupied.
- If a cell is unoccupied and exactly 3 of the 8 adjacent cells are occupied, a new bacteria is born, and the cell becomes occupied.
Details
Create a file called game_of_life.py
to play
Conway's Game of Life. The program should load a board, a
two-dimensional list of 0s and 1s, from a file and repeatedly print
the result of updating the board according to the above rules. The
program should end when the user kills the program by pressing
ctrl-c.
The first line of the input file is going to be a specification of the number of rows (m)in the board and the number of columns (n) in the board, separated by a single space. These tell you the number of lines to follow, and the length of each line. The rest of the input file should be formatted as an m \times n grid of 1's and 0's.
Example
If the input file looks like this:
11 11 00000000000 00000000000 00000000000 00000000000 00000100000 00001110000 00000100000 00000000000 00000000000 00000000000 00000000000
The program output should be:
00000000000 00000000000 00000000000 00000000000 00000100000 00001110000 00000100000 00000000000 00000000000 00000000000 00000000000 00000000000 00000000000 00000000000 00000000000 00001110000 00001010000 00001110000 00000000000 00000000000 00000000000 00000000000 00000000000 00000000000 00000000000 00000100000 00001010000 00010001000 00001010000 00000100000 00000000000 00000000000 00000000000
Hint
Challenge
As you have no doubt seen, watching The Game of Life through the terminal window is incredibly difficult. The 1's and 0's flying past the screen do very little to aid in your visual of what is going on. Let's layer a Graphical Interface on top of your 2-d list representation, which hopefully will make it easier to visualize.
Create a new function called draw_game
. This
function should take the game board as a parameter, plus any
additional information necessary to perform the drawing. You
should be able to simply call draw_game
in the same
place you have been calling print_game
, with minimal
changes else where.
Your visual should consist of a 2-d grid, where each cell in the grid represents one of the values from the 2-d list of values representing the game board. Cells that are valued 0 will not be filled in, while cells that are valued 1 will be filled in with a color of your choosing (traditionally black).
Your grid should fill as much of the window as possible. As such, you should decide the size of the cells based off the dimensions of the window, as opposed to specifying a default grid size.
Example
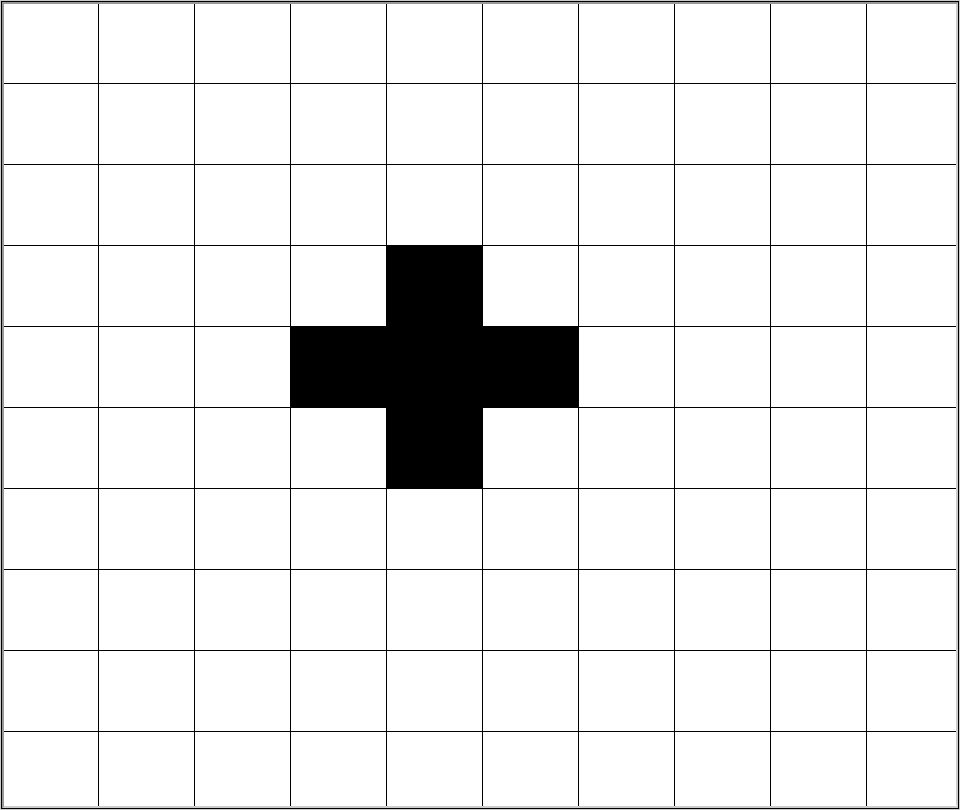
Submission
Please show your source code and run your programs for the instructor or lab assistant. Only a programs that have perfect style and flawless functionality will be accepted as complete.