6.2. Function Parameters¶
Recall the main function from the last section that draws a square:
It is not any better than a program that draws a square, without a function. In fact, it is probably worse because it includes lots of code that is not necessary for drawing a square. To make functions more useful we need to create functions that don’t always do the same thing. We need function with input. For example, the draw square function would be more useful if you could specify the size of the square it draws. The following is a new draw square function that has input:
The inputs to a function, or the parameters, are listed inside the
parenthses after the function name. Parameter definitions are similar
to variable definitions. They are the parameter name and type
separated with a colon. In the above draw_square
function, there
is one parameter, called size, with type float.
The value of a parameter is specified when the function is called. In
the above example, the draw_square
function is called on line 10
and it specifies a value of 50.0
for the size
parameter inside
of the parentheses.
Think of parameters like variables, except you do not specify the value of the parameters anywhere inside the function. Instead the parameter values are specified when the function is called.
The figure below shows this relationship. A function needs certain information to do its work. These values, often called arguments are passed to the function by the user.
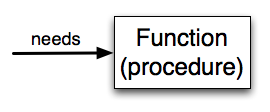
This type of diagram is often called a black-box diagram because it only states the requirements from the perspective of the user. The user must know the name of the function and what arguments need to be passed. The details of how the function works are hidden inside the “black-box”.
Suppose we’re working with turtles and a common operation we need is to draw squares. It would make sense if we did not have to duplicate all the steps each time we want to make a square. “Draw a square” can be thought of as an abstraction of a number of smaller steps. We will need to provide one piece of information for the function to do its work: a size for the side of the square. We could represent this using the following black-box diagram.
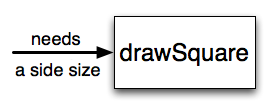
Once we’ve defined a function, we can call it as often as we like and
its statements will be executed each time we call it. In this case,
we could use it to get one of our turtles to draw a square and then we
can move the turtle and have it draw a different square in a different
location. Note that we lift the tail so that when the turtle moves
there is no trace. We put the tail back down before drawing the next
square. Make sure you can identify both invocations of the
draw_square
function.
There are a few restrictions on the use of functions that are worth knowing:
Variable declarations in a function must be before all other code.
Functions can not contain import statements.
The last line of a function must be return statement (
return None
for now)Functions must be defined above the function from which they are called.
The main function is a special function with it’s own set of rules:
Any program that defines a function must define the main function.
The main function is the only function that can be called from outside any function.
The call to the main function must be the last line in the program.
Warning
Even if a function call needs no arguments, the parentheses ( )
after the function name are required. This can lead to a
difficult bug: A function name without the parenthesis is a legal
expression referring to the function; for example, print
and
turtle.setposition
, but they do not call the associated
functions.
Check your understanding
- def draw_circle() -> None:
- A function may take zero or more parameters. It does not have to have any. In this case the size of the circle might be specified in the body of the function.
- def draw_circle -> None:
- A function needs to specify its parameters in its header.
- draw_circle(size: float) -> None:
- A function definition needs to include the keyword def.
- def draw_circle(size:float) -> None
- A function definition header must end in a colon (:).
func-1-1: Which of the following is a valid function header (first line of a function definition)?
- def draw_square(size: float) -> None
- This line is the complete function header.
- draw_square
- Yes, the name of the function is given after the keyword def and before the list of parameters.
- draw_square(size: float)
- This includes the function name and its parameters
- Make turtle draw a square with side size.
- This is a comment stating what the function does.
func-1-2: What is the name of the following function?
# Make turtle draw a square with side size
def draw_square(size: float) -> None:
for i in range(0, 4, 1):
turtle.forward(size)
turtle.left(90.0)
return None
- i
- i is a variable used inside of the function, but not a parameter, which is passed in to the function.
- size
- size is only one of the parameters to this function.
- size, color
- Yes, the function specifies two parameters: size and color.
- size, color, i
- the parameters include only those variables whose values that the function expects to receive as input. They are specified in the header of the function.
func-1-3: What are the parameters of the following function?
def draw_square(size: float, color: str) -> None:
turtle.fillcolor(color)
turtle.begin_fill()
for i in range(0, 4, 1):
turtle.forward(size)
turtle.left(angle)
turtle.end_fill()
return None
- def draw_square(size, color)
- No, size and color are the names of the formal parameters to this function. When the function is called, it requires actual values to be passed in.
- draw_square
- A function call always requires parentheses after the name of the function.
- draw_square(10.0)
- This function takes two parameters (arguments)
- draw_square(10.0, 'red'):
- A colon is only required in a function definition. It will cause an error with a function call.
- draw_square(10.0, 'red')
- Since 10.0 and 'red' are values, we have passed in two correct values for this function.
func-1-4: Considering the function below, which of the following statements correctly invokes, or calls, this function (i.e., causes it to run)?
def draw_square(size: float, color: str) -> None:
turtle.fillcolor(color)
turtle.begin_fill()
for i in range(0, 4, 1):
turtle.forward(size)
turtle.left(angle)
turtle.end_fill()
return None