Curve
Computer displays are made up of a grid of tiny lights. Because the displays are not continuous, curved objects must be broken into many non-continuous pieces. So curves are often simply represented as many straight lines. In this activity you will approximate a curve by drawing many lines.
Details
Create a Python program that uses the turtle module to draw a curve using overlapping straight lines between the x-axis and the y-axis. The program should prompt the user to enter the number of lines to draw and the length of the axes.
Example
Enter the number of lines: 5 Enter the length of the axes: 100
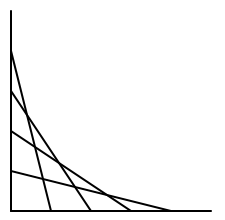
Enter the number of lines: 50 Enter the length of the axes: 100
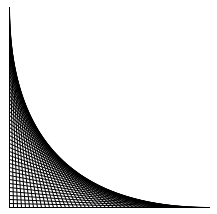
- You want to use the new range values for this activity. You need to determine how much space to be between consecutive points on the lines. This will be the step amount for your loop.
-
Drawing the curve is much easier if you use the
function
turtle.setposition
. - For each line, calculate the coordinates of both end points
-
Use the
turtle.setposition
function to move the turtle to the start point, then use theturtle.setposition
function again to move the turtle to the end point. -
Note that you may need to use the
turtle.penup
andturtle.pendown
functions to prevent the turtle from drawing a line when it moves to the line's start end point.
Challenge
This same technique can be used to draw a myriad of different curves, by changing the length and angle of the axes. Add variable to your program that specify the length and angle of each of the axes to create curves like the following.
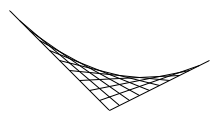
You can even put the code that draws the curve in a loop to draw multiple curves.
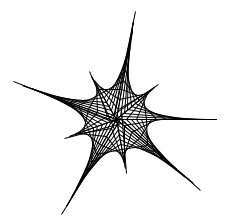