Leibniz Approximation
The mathematical constant π is an irrational number that represents the ratio between a circle's diameter and its circumference. The first algorithm designed to approximate π was developed by Archimedes in 250 BC, and was able to approximate its value within 3 significant figures. Today we know that there are an infinite number of digits in π, so lets use a little more sophisticated approach to estimate its value.
Details
Create that prompts the user to input an integer, which is the number of terms to compute in the infinite series. It should use this value to compute the Leibniz approximation, discussed below.
The Leibniz approximation relies on the fact that:
π4=11−13+15−17+19−…
Which can be rewritten to approximate the true value of π:
π=41−43+45−47+49−…
Example
- You will need a for loop that uses the accumulator pattern to accomplish this goal. Create a variable (defaulted value to 0) before the for loop. Then you just need to update the value of the variable inside the for loop.
- As you progress through your for loop, the denominator of your fraction increases by a factor of two. Some simple algebra will reveal that during loop iteration i, the denominator is: (2×i)+1
- You need to alternate adding and subtracting. However, subtracting is the same as adding a negative number. So we can accomplish this by multiplying every other fraction by -1. The easy way to do this is to compute: (−1)i Where i is your loop variable.
- The accumulator is the sum of all of the terms in the sequence, so you only need to add one term to the accumulator during each loop iteration.
Challenge
The value you get from the Leibniz formula will be incredibly close.
However, it will never meet the true definition of π. To see how
close it does get, compute the approximations
for all equations from length 1 to length 1000.
For each approximation, subtract the value of
pi, 3.141592653589793
, from it to compute the error.
Sum up all of these errors, and print the average error.
Fish
Since we have a test coming up, lets do a little bit of a fun activity. One of the badges of honor for the CS major comes from this activity: drawing a fish. I've had alumni tell me that the activity they remember from this class is the fish. Hopefully they remember if fondly.
Details
Create a Python program that prompts the user to enter two integers, which are the location on the turtle window to draw a line drawing of a fish. Your fish should be drawn centered on the specified coordinate.
Start on paper first!. The activity will progress much easier for you if you figure out the coordinates of various points before you begin coding. Start with a plan and your code will come together very quickly.
Example
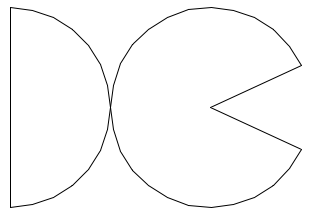
While this looks very complicated, it's just two partial circles and three lines. You just need to figure out the locations of certain points before you get started. Consider the following image if you need assistance:
Where the fish is 3 × r pixels wide from tail to the tip of the head.
Challenge
What do you call a fish with no eyes? FSH! Add eyes to your fish so it won't accidentally swim into a dam!