2.4. Variables¶
One of the most powerful features of a programming language is the ability to manipulate variables. A variable is a name that refers to a value.
Creating a variable consists of two steps, declaration and assignment.
Declaration statements associate the name and type of a variable.
message: str
n: int
pi: float
This example declares three variables. The first is named message
and is a string type. The second is named n
and is an integer type. The third is named pi
and is a floting-point type.
Note
All variable declarations must come after any import statements and before any other lines of code in a program.
Assignment statements create new variables and also give them values to refer to.
message: str
n: int
pi: float
message = "What's up, Doc?"
n = 17
pi = 3.14159
This example makes three assignments. The first assigns the string value
"What's up, Doc?"
to the variable named message
. The second gives the
integer 17
to n
, and the third assigns the floating-point number
3.14159
to a variable called pi
.
The assignment token, =
, should not be confused with equality (we will see later that equality uses the
==
token). The assignment statement links a name, on the left hand
side of the operator, with a value, on the right hand side. This is why you
will get an error if you enter:
n: int
17 = n
Tip
When reading or writing code, say to yourself “n is assigned 17” or “n gets the value 17” or “n is a reference to the object 17” or “n refers to the object 17”. Don’t say “n equals 17”.
A common way to represent variables on paper is to write the name with an arrow pointing to the variable’s value. This kind of figure, known as a reference diagram, is often called a state snapshot because it shows what state each of the variables is in at a particular instant in time. (Think of it as the variable’s state of mind). This diagram shows the result of executing the assignment statements shown above.
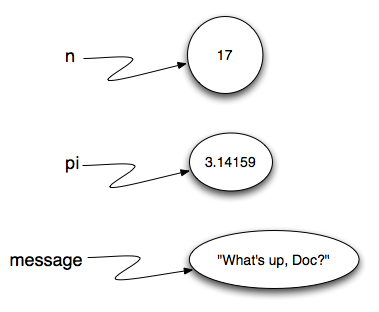
If you ask Python to evaluate a variable, it will produce the value that is currently linked to the variable. In other words, evaluating a variable will give you the value that is referred to by the variable.
In each case the result is the value of the variable. To see this in even more detail, we can run the program using codelens.
(ch02_9_codelens)
Now, as you step through the statements, you can see the variables and the values they reference as those references are created.
The value that a variable is assigned must match the type that is assigned to it. The following program will produce an error:
Check your understanding
- Nothing is printed. A runtime error occurs.
- The type of the variable and the type of the assignment value match so there is no runtime error.
- Thursday
- The variable day is assigned the value 'Thursday' before being printed.
- 32.5
- The variable day is not assigned the value 32.5.
- 19
- The variable day is not assigned the value 19.
data-4-1: What is printed when the following statements execute?
day: str
day = "Thursday"
print(day)
- Nothing is printed. A runtime error occurs.
- The type of the variable and the type of the assignment value do not match.
- Thursday
- The variable day is not assigned the value 'Thursday'.
- 32.5
- The variable day is not assigned the value 32.5.
- 19
- The variable day is declared to be a string type, so assigning an integer to it will cause an error.
data-4-2: What is printed when the following statements execute?
day: str
day = 19
print(day)