Average
Write a python program that reads 4 numbers from the user and prints the average of the numbers. Note, this program could be written without reusing or updating any variables, but for the sake of practice, write the program using only one variable to compute the sum.
Example
Enter a number: 1.0 Enter a number: 2.0 Enter a number: 3.0 Enter a number: 4.0 The average is 2.5
Spiral
The human visual system did not evolve to percieve the types of regular patterns that are simple to create with programming. A spiral of contrasting colors can produce the illusion of motion when you move your eyes over the static image.
Details
Create a Python program that uses turtle graphics to draw a square spiral. The program should prompt the user to enter the amount that each line is longer than the previous line that was drawn. The program can have any number of lines, but more lines look cooler. Again, you can write this program without variable updating, but for the sake of practice, write it using using a single variable for the distance that the turtle moves that is repeatedly updated.
Example
Enter the line increase amount: 10
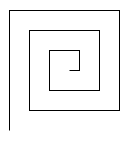
- To draw the square spiral the turtle should repeatedly move forward and turn.
- The turtle should always turn 90°.
- The distance forward the turtle moves should increase with each line. Create a variable for the distance the turtle moves and update the value of the variable before drawing each line. Use the value entered by the user as the amount the variable increases by.
Challenge
Instead of a spiral create, create concentric squares.
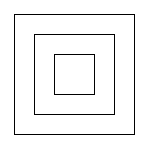
World's Largest Ice Cream Cone
The world's largest ice cream cone was 9 feet tall, according to the Guinness Book of World Records. While impressive based on the height alone, one has to wonder how much ice cream actually went into making such a monstrosity. Math is of course our friend, but writing a program could make it easier to figure out how big of an ice cream cone you and your friends would have to make in order to beat the current record.
Details
Create a Python program that prompts the user to enter the height and radius of an ice cream cone, in feet, and prints the volume, in cubic feet. Assume that the entire cone is filled with ice cream and that there is a perfect half-sphere of ice cream on top of the cone, like this:
The volume of a cone can be computed using the equation:
v=π⋅r2⋅h3
Where v is the volume of a cone, r is the radius of the cone, and h is the height of the cone. The volume of a sphere can be computed using the equation:
v=43⋅π⋅r3
Where v is the volume of a sphere and r is the radius of the sphere. The program should use meaningful names for values and simple statements to make the program more readable. The output should be nicely formatted and labeled.
Example
Enter the height of the cone in feet: 9.0 Enter the radius of the cone in feet: 2.0 The volume of the cone is 37.69911184307752 cubic feet. The volume of the sphere is 33.510321638291124 cubic feet. The volume of ice cream is 54.454272662223076 cubic feet.
- First, create variables for the input values to the program.
In this case
cone_height
andcone_radius
are the inputs to the program. Don't forget to convert the input from a string to a float! - Next, create variables for the computations that will be
performed. In this
case
cone_volume
,sphere_volume
, andice_cream_volume
. Set each of these variables equal to arithmetic expressions that compute the correct value. Note that theice_cream_volume
variable depends on the other two variables, so it must be defined after them. - Finally, create print statements that print the calculated values nicely formatted.
Challenge
So how many children can the world's largest ice cream cone feed? Modify your program to calculate and print the result. Assume that a single serving of ice cream is 4 oz and note that there are 957.506 oz in a cubic foot.