11.2. Finding a File on your Disk¶
Opening a file requires that you, as a programmer, and Python agree about the
location of the file on your disk. The way that files are located on disk is
by their path You can think of the filename as the short name for a file,
and the path as the full name. For example on a Mac if you save the file
hello.txt
in your home directory the path to that file is
/Users/yourname/hello.txt
On a Windows machine the path looks a bit different
but the same principles are in use. For example on windows the path might be
C:\Users\yourname\My Documents\hello.txt
You can access files in sub-folders, also called directories, under your home directory
by adding a slash and the name of the folder. For example, if you had a file
called hello.py
in a folder called CS150
that is inside a folder called
PyCharmProjects
under your home directory, then the full name for the file
hello.py
is /Users/yourname/PyCharmProjects/CS150/hello.py
.
This is called an absolute file path. An absolute file path typically
only works on a specific computer. Think about it for a second. What other
computer in the world is going to have an absolute file path that starts with
/Users/yourname
?
If a file is not in the same folder as your python program, you need to tell
the computer how to reach it. A relative file path starts from the folder
that contains your python program and follows a computer’s file hierarchy. A
file hierarchy contains folders which contains files and other sub-folders.
Specifying a sub-folder is easy – you simply specify the sub-folder’s name.
To specify a parent folder you use the special ..
notation because every file
and folder has one unique parent. You can use the ..
notation multiple times in a file path to move multiple levels up a file
hierarchy. Here is an example file hierarchy that contains multiple folders,
files, and sub-folders. Folders in the diagram are displayed in bold type.
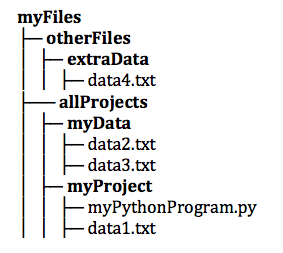
Using the example file hierarchy above, the program, myPythonProgram.py
could access each of the data files using the following relative file paths:
data1.txt
../myData/data2.txt
../myData/data3.txt
../../otherFiles/extraData/data4.txt
Here’s the important rule to remember: If your file and your Python program are
in the same directory you can simply use the filename like this:
open('myfile.txt', 'r')
. If your file and your Python program are in
different directories then you must use a relative file path to the file
like this: open('../myData/data3.txt', 'r')
.
11.2.1. Glossary¶
- absolute file path
- The name of a file that includes a path to the file from the root
directory of a file system. An absolute file path always starts
with a
/
. - relative file path
- The name of a file that includes a path to the file from the current
working directory of a program. An relative file path never starts
with a
/
.