Graphics¶
Make drawing, animating, and creating games as easy as using Turtle.
Download the graphics.py
file to use
the module.
Drawing Functions¶
-
graphics.
draw_image
(image_name, x, y)¶ Draw the specified image to the Graphics window.
Parameters: - image_name (str) – The file name of the image to draw. The image must be a GIF file.
- x (float) – The x-coordinate, in pixels, of the center of the image to draw.
- y (float) – The y-coordinate, in pixels, of the center of the image to draw.
See Coordinate System for a description of the Graphics window coordinate system.
-
graphics.
draw_sprite
(sprite)¶ Draw the specified sprite to the Graphics window.
Parameters: sprite (Sprite) – The sprite object to draw.
-
graphics.
image_size
(image_name)¶ Return the size of the specified image when drawn to the Graphics window.
Parameters: image_name (str) – The file name of the image to draw. Returns: (int, int) – The width and height of the image as a tuple of integers. If
image_name
is not a valid image file (0, 0) is returned.
-
graphics.
draw_text
(text, x, y, color='black', size=12)¶ Draw the specified text to the Graphics window.
Parameters: - text (str) – The text to draw to the window.
- x (float) – The x-coordinate, in pixels, of the center of the text to draw.
- y (float) – The y-coordinate, in pixels, of the center of the text to draw.
- color (str) – The color of the text to draw. Default value is ‘black’.
- size (int) – The size, in points, of the text to draw. Default value is 12.
See Coordinate System for a description of the Graphics window coordinate system.
See TKinter Colors Strings for a description of valid TKinter color strings.
-
graphics.
text_size
(text, size=12)¶ Return the size of the specified text when drawn to the Graphics window.
Parameters: - text (str) – The text to get the size of.
- size (int) – The size, in points, of the text to get the size of.
Returns: (int, int) – The width and height of the text as a tuple of integers.
-
graphics.
clear
()¶ Clear all images and text drawn to the Graphics window.
Returns: (float) – The number of seconds since the last time this function was called.
-
graphics.
mainloop
()¶ Keep the Graphics window open.
This function is to be used to keep the program from ending when no animation is being done, aka displaying a drawing. This will keep the Graphics window open until the user closes the window, either by hitting the escape key or by clicking on the window close button, or until
window_open(False)
is called.- For example::
>>> draw_something_brilliant() # you must supply this function >>> mainloop() # window will stay open
Window Functions¶
-
graphics.
window_size
(width=None, height=None)¶ Return or set size of the Graphics window.
Parameters: - width (int) – The width of the window drawing area, in pixels.
- height (int) – The height of the window drawing area, in pixels.
Returns: (int, int) – The current width and height of the window as a tuple of integers.
- For example::
>>> graphics.window_size(640, 480) # the drawing area will be 640 x 480 >>> print(graphics.window_size()) >>> (640, 480) >>> graphics.window_size(width=480) # the drawing area width will be 480 >>> print(graphics.window_size()) >>> (480, 480)
-
graphics.
window_title
(title=None)¶ Return or set the title of the Graphics window.
Parameters: title (str) – The title of the Graphics window. Returns: (str) – The current window title. - For example::
>>> graphics.window_title('Mine') # window title will be 'Mine' >>> print(graphics.window_title()) >>> Mine >>> graphics.window_title('Not Yours') # window title will be 'Not Yours' >>> print(graphics.window_title()) >>> Not Yours
-
graphics.
window_background_color
(color=None)¶ Return or set the background color of the Graphics window.
Parameters: color (str) – The color to set the background of the window to. Returns: (str) – The current window background color. - See TKinter Colors Strings for a description of valid TKinter color
- strings.
- For example::
>>> graphics.window_background_color('black') # black window background >>> print(graphics.window_background_color()) >>> black >>> graphics.window_background_color('white') # white window background >>> print(graphics.window_background_color()) >>> white
-
graphics.
frame_rate
(frames_per_second=None)¶ Return or set the frames-per-second of the Graphics window.
Parameters: frames_per_second (int) – The frames-per-second to set the Graphics window to. Returns: (bool) – The current window frames-per-second. The frames-per-second of the Graphics window is the minimum amount of time between two calls to the update function. This is useful in creating animation with a constant frame rate. Note, the the amount of time may be more than the frames-per-second if the drawing is too complex or the computer is too slow.
- For example::
>>> graphics.frame_rate(30) # window updates at most 30 times per second >>> print(graphics.frame_rate()) >>> 30 >>> graphics.frame_rate(60) # window updates at most 60 times per second >>> print(graphics.frame_rate()) >>> 60
-
graphics.
window_open
(win_open=None)¶ Return or set whether the Graphics window is open.
Parameters: win_open (bool) – Whether to open or close the window. Returns: (bool) – Whether the window is open or closed. - For example::
>>> graphics.window_open(True) # The window will open if it isn't already >>> print(graphics.window_open()) >>> True >>> graphics.window_open(False) # The window will close if it isn't already >>> print(graphics.window_open()) >>> False
Keyboard Functions¶
-
graphics.
key_down
(key_symbol)¶ Return whether the specified keyboard key is currently down.
Parameters: button_symbol (str) – A valid TKinter key symbol string. Returns: (bool) – True if the specified keyboard key is being pressed, false otherwise. See TKinter Key Symbols for a list of valid key symbols.
-
graphics.
key_up
(key_symbol)¶ Return whether the specified keyboard key is currently up.
Parameters: button_symbol (str) – A valid TKinter key symbol string. Returns: (bool) – True if the specified keyboard key is not being pressed, false otherwise. See TKinter Key Symbols for a list of valid key symbols.
-
graphics.
key_pressed
(key_symbol)¶ Return whether the specified keyboard key was pressed.
Parameters: key_symbol (str) – A valid TKinter key symbol string. Returns: (bool) – True if the specified keyboard key was pressed, false otherwise. See TKinter Key Symbols for a list of valid key symbols.
Note, this function returns whether the specified key was pressed since the last time the clear function was called. It is meant to be used in a program with an active loop that calls the clear function every iteration.
-
graphics.
key_released
(key_symbol)¶ Return whether the specified keyboard key was released.
Parameters: key_symbol (str) – A valid TKinter key symbol string. Returns: (bool) – True if the specified keyboard key was released, false otherwise. See TKinter Key Symbols for a list of valid key symbols.
Note, this function returns whether the specified key was released since the last time the clear function was called. It is meant to be used in a program with an active loop that calls the clear function every iteration.
-
graphics.
wait_for_key_press
()¶ Waits for the user to press a key and returns the key symbol.
Returns: (str) – The TKinter key symbol string of the key pressed by the user. See TKinter Key Symbols for a list of key symbols.
Note, this is a blocking function. When invoked, the calling program will stop until the user presses a keyboard key.
-
graphics.
wait_for_key_release
()¶ Waits for the user to release a key and returns the key symbol.
Returns: (str) – The TKinter key symbol string of the key released by the user. See TKinter Key Symbols for a list of key symbols.
Note, this is a blocking function. When invoked, the calling program will stop until the user releases a keyboard key.
-
graphics.
wait_for_text
(x, y, foreground='black', background='white', size=12, width=20)¶ Waits for the user to type text into text box and returns text
Parameters: - x (float) – The x-coordinate, in pixels, of the center of the text box.
- y (float) – The y-coordinate, in pixels, of the center of the text box.
- foreground (str) – The color of the text in the text box. Default value is ‘black’.
- background (str) – The color of the background of the text box. Default value is ‘white’.
- size (int) – The font size, in points, of the text in the text box. Default value is 12.
- width (int) – The width, in characters, of the text box. Default value is 20.
Returns: (str) – The text entered by the user.
Note, this is a blocking function. When invoked, the calling program will stop until the user presses the return key.
Mouse Functions¶
Return whether the specified mouse button is currently down.
Parameters: button_symbol (int) – A valid TKinter button number. Returns: (bool) – True if the specified mouse button is being pressed, false otherwise. See TKinter Button Numbers for a list of valid button numbers.
Return whether the specified mouse button is currently up.
Parameters: button_number (int) – A valid TKinter button number. Returns: (bool) – True if the specified mouse button is not being pressed, false otherwise. See TKinter Button Numbers for a list of valid button numbers.
Return whether the specified mouse button was pressed.
Parameters: button_number (int) – A valid TKinter button number. Returns: (bool) – True if the specified mouse button was pressed, false otherwise. See TKinter Button Numbers for a list of valid button numbers.
Note, this function returns whether the specified button was pressed since the last time the clear function was called. It is meant to be used in a program with an active loop that calls the clear function every iteration.
Return whether the specified mouse button was released.
Parameters: button_number (int) – A valid TKinter button number. Returns: (bool) – True if the specified mouse button was released, false otherwise. See TKinter Button Numbers for a list of valid button numbers.
Note, this function returns whether the specified button was released since the last time the clear function was called. It is meant to be used in a program with an active loop that calls the clear function every iteration.
Waits for the user to press a mouse button and returns the number.
Returns: (int) – The TKinter mouse button number of the mouse button pressed by the user. See TKinter Button Numbers for a list of button numbers.
Note, this is a blocking function. When invoked, the calling program will stop until the user presses a mouse button.
Waits for the user to release a mouse button and returns the number.
Returns: (int) – The TKinter mouse button number of the mouse button released by the user. See TKinter Button Numbers for a list of button numbers.
Note, this is a blocking function. When invoked, the calling program will stop until the user releases a mouse button.
-
graphics.
mouse_x
()¶ Return the x coordinate of the mouse’s current location.
-
graphics.
mouse_y
()¶ Return the y coordinate of the mouse’s current location.
-
graphics.
mouse_moved
()¶ Return whether the mouse moved.
Note, this function returns whether the mouse moved since the last time the clear function was called. It is meant to be used in a program with an active loop that calls the clear function every iteration.
Coordinate System¶
The point (0, 0) is the upper left of the window. Positive x is to the right and positive y is down. For example:
>>> import graphics
>>> window_width = 160
>>> window_height = 120
>>> graphics.window_size(window_width, window_height)
>>> graphics.window_background_color('gray')
>>> graphics.draw_image('red_circle.gif', 0, 0)
>>> graphics.draw_image('green_circle.gif', window_width, 0)
>>> graphics.draw_image('blue_circle.gif', 0, window_height)
>>> graphics.mainloop()
Would produce the following drawing.
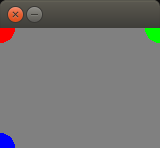
The red circle is at (0, 0), the green circle is at (160, 0), and the blue circle is at (0, 120).
TKinter Colors Strings¶
The following are valid TKinter Color Strings:
Color Strings | |||||||
---|---|---|---|---|---|---|---|
snow | mint cream | cornflower blue | light blue | sea green | pale goldenrod | light salmon | medium violet red |
ghost white | azure | dark slate blue | powder blue | medium sea green | light goldenrod yellow | orange | violet red |
white smoke | alice blue | slate blue | pale turquoise | light sea green | light yellow | dark orange | medium orchid |
gainsboro | lavender | medium slate blue | dark turquoise | pale green | yellow | coral | dark orchid |
floral white | lavender blush | light slate blue | medium turquoise | spring green | gold | light coral | dark violet |
old lace | misty rose | medium blue | turquoise | lawn green | light goldenrod | tomato | blue violet |
linen | dark slate gray | royal blue | cyan | medium spring green | goldenrod | orange red | purple |
antique white | dim gray | blue | light cyan | green yellow | dark goldenrod | red | medium purple |
papaya whip | slate gray | dodger blue | cadet blue | lime green | rosy brown | hot pink | thistle |
blanched almond | light slate gray | deep sky blue | medium aquamarine | yellow green | indian red | deep pink | |
bisque | gray | sky blue | aquamarine | forest green | saddle brown | pink | |
peach puff | light grey | light sky blue | dark green | olive drab | sandy brown | light pink | |
navajo white | midnight blue | steel blue | dark olive green | dark khaki | dark salmon | pale violet red | |
lemon chiffon | navy | light steel blue | dark sea green | khaki | salmon | maroon |
TKinter Key Symbols¶
The following are valid TKinter key symbols:
Key Symbols | ||||||||||
---|---|---|---|---|---|---|---|---|---|---|
space | slash | greater | M | backslash | k | z | Kanji | Execute | F6 | Super_L |
exclam | 0 | question | N | bracketright | l | braceleft | Home | Insert | F7 | Super_R |
quotedbl | 1 | at | O | asciicircum | m | bar | Left | Undo | F8 | Hyper_L |
numbersign | 2 | A | P | underscore | n | braceright | Up | Redo | F9 | Hyper_R |
dollar | 3 | B | Q | quoteleft | o | asciitilde | Right | Menu | F10 | Delete |
percent | 4 | C | R | a | p | BackSpace | Down | Find | Shift_L | |
ampersand | 5 | D | S | b | q | Tab | Prior | Cancel | Shift_R | |
quoteright | 6 | E | T | c | r | Linefeed | Next | Help | Control_L | |
parenleft | 7 | F | U | d | s | Clear | End | Break | Control_R | |
parenright | 8 | G | V | e | t | Return | Begin | Num_Lock | Caps_Lock | |
asterisk | 9 | H | W | f | u | Pause | Win_L | F1 | Shift_Lock | |
plus | colon | I | X | g | v | Scroll_Lock | Win_R | F2 | Meta_L | |
comma | semicolon | J | Y | h | w | Sys_Req | App | F3 | Meta_R | |
minus | less | K | Z | i | x | Escape | Select | F4 | Alt_L | |
period | equal | L | bracketleft | j | y | Multi_key | F5 | Alt_R |
TKinter Button Numbers¶
The following are valid TKinter button numbers:
Button Number | Mouse Button |
---|---|
1 | Left Mouse Button |
2 | Middle Mouse Button |
3 | Right Mouse Button |
4 | Up Mouse Scroll Wheel |
5 | Up Mouse Scroll Wheel |
Examples¶
Here is an example of drawing using the Graphics module:
import graphics
# Initialize the window
window_width = 640
window_height = 480
window_background_color = 'white'
window_title = 'Testing...'
graphics.window_size(window_width, window_height)
graphics.window_background_color(window_background_color)
graphics.window_title(window_title)
# Draw a circle to it
circle_image_name = 'red_circle.gif'
circle_x = 200
circle_y = 100
graphics.draw_image(circle_image_name, circle_x, circle_y)
# Draw some text on top of the circle
text = 'Testing'
text_x = circle_x
text_y = circle_y
text_color = 'black'
text_size = 12
graphics.draw_text(text, circle_x, circle_y, text_color, text_size)
# Keep the window from closing after drawing
graphics.mainloop()
Here is an example of animating using the Graphics module:
import graphics
# Initialize the window
window_width = 640
window_height = 480
window_background_color = 'white'
window_title = 'Testing...'
graphics.window_size(window_width, window_height)
graphics.window_background_color(window_background_color)
graphics.window_title(window_title)
# Initialize a circle
circle_image_name = 'red_circle.gif'
circle_x = 200
circle_y = 100
number_of_frames = 100
# Animate the circle for a fixed number of frames
for frame in range(number_of_frames):
# Erase any previous drawing
graphics.clear()
# Change the location of the circle
circle_x += 1
# Draw the circle at the new location
graphics.draw_image(circle_image_name, circle_x, circle_y)
# Keep the window from closing after animating
graphics.mainloop()
Here is an example of user interaction using the Graphics module:
import graphics
# Initialize the window
window_width = 640
window_height = 480
window_background_color = 'white'
window_title = 'Testing...'
graphics.window_size(window_width, window_height)
graphics.window_background_color(window_background_color)
graphics.window_title(window_title)
# Initialize a circle
circle_image_name = 'red_circle.gif'
circle_x = 200
circle_y = 100
number_of_frames = 100
# Animate the circle until the window closes
while graphics.window_open():
# Erase any previous drawing
graphics.clear()
# Change the location of the circle based on what key the user is pressing
if graphics.key_down('Right'):
circle_x += 1
if graphics.key_down('Left'):
circle_x -= 1
# Draw the circle at the new location
graphics.draw_image(circle_image_name, circle_x, circle_y)
# Keep the window from closing after animating
graphics.mainloop()