CPSC 120 Fall 2007
Assignnment 6: Color Gradients
Due Friday, December 7, by 4 pm
Color Gradients
Color Gradients are a visual technique that blend two colors
together; transitioning from one color to the another over a fixed
space. The images below show some of the ways that Color
Gradients can be used to achieve various visual effects.
A) blends a bold color (blue) with the background color
(white). The effect is that the bottom boundary of
the box has faded away, while the top boundary is crisp and well
defined. B) blends two colors to produce an effect that may
resemble a sunrise. C) The word 'Hot' is reinforced by
blending together colors reminiscent of fire. D)
Blending colors that are slightly brighter and slightly darker than the
background can produce a 3D effect.
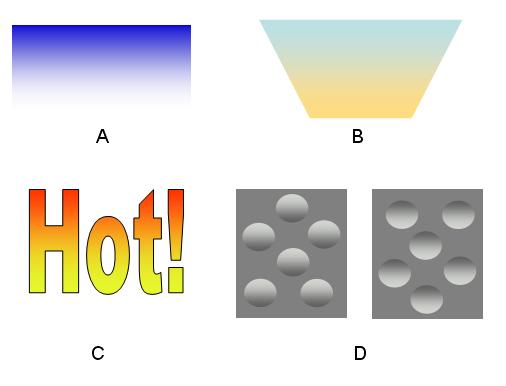
For this assignment, you will create a program that allows the user to
select colors and blend them together to see their
gradient.
Program Interface
Your
program should display color menus at the top and bottom of the window.
When the user clicks on a color in either menu a gradient between that
color and the one in the other menu
should be displayed in the space between the menus.
When a different color is clicked on in either
menu, the corresponding color in the gradient should change.
Each menu should have at least five color buttons. Additionally,
there should be a set of text boxes that allow the user to
specify a "Custom Color" button. Remember that the
Color class can specify colors in terms of Red, Green and Blue
components (so you will need three text boxes as well as the
corresponding button). A demo will be posted on the
course web page to give you an idea of what the GUI should look like.
Program Design and Specifications
To complete this program, you will need to create three
files: ColorButtonPanel.java, GradientPanel.java, and ColorGradientApp.java
ColorButtonPanel:
This class is responsible for creating a menu of Buttons that allows the user
to select the color to be used in the gradient.
It will consist of the following instance data:
- At least 5 JButton objects to represent the colors(4
default + 1 custom)
- At least 5 Color objects: Each button will have a color object
associated with it.
- A Color object to store the color of the button that was pushed
last.
- Three JTextFields to enter in the color components for the
customized color and their corresponding labels.
- 2 ints to store the overall menu width and height in pixels.
- A GradientPanel object - needed to tell what panel
should be repainted when a new
color when a button is pressed.
The following public methods are also needed:
- A constructor -- takes the default starting colors and a
GradientPanel and sets up the button panel (& the listeners
needed)
. Recall that all Java components have the methods
setBackground for setting the background color of the component
and setPreferredSize for setting the size of the component.
- getSelectedColor -- returns a color object
associated with the button
that was last selected
The ColorButtonPanel class should have a nested listener class to
listen for and respond to button clicks.
When the Custom button is pushed the
listener should get the red, green, and blue components from the
text fields and create the current color.
GradientPanel: This class is the main panel
where the gradients will be drawn
The following
instance variables are needed.
- Two ColorButtonPanels: one for the top, one for the
bottom. Note that the constructors for ButtonPanels take a
GradientPanel object. Since you are calling the constructor from inside
of the GradientPanel class, you can use the keyword this to pass "this" object
into ColorButtonPanel's constructor.
- int width, height: the overall panel width and height
in
pixels
- int panelHeight: a variable to track the height of the
Gradient Panel that is eligible for drawing.
The GradientPanel class also needs to provide the following
public methods:
- A constructor -- sets up the components
- paintComponent(Graphics page): The method should
redraw the gradient to the screen. At a broad level, this
is accomplished by starting at the top of the viewing region and
repeatedly drawing horizontal
lines across the screen until the viewing region is
filled. Each time a line is drawn, the color changes
slightly from the color at the top of the screen until it is completely
transformed into the ending color at the bottom of the screen.
Some good questions to think about:
- How many lines will be drawn?
- How much different is the top color from the bottom
color? (Remember, colors are represented in terms of Red, Green and
Blue components, so you probably want to ask How different in
Red?
How different in Green? and How different in Blue?).
- If I know how different the first and last lines need to be
and I know how many lines there are going to be, can I figure out how
different each line is going to be? (in terms of Red, Green and
Blue). Note: even though the
difference in color (RGB values) are expressed in whole numbers, the
difference from line to line may be fractional! Consider
what happens if there are 100 lines to be drawn, but a color
differential of 20.
ColorGradientApp: Contains the main
method of the application - Creates a JFrame that contains a
GradientPanel.
Implementation Strategy
As always, you should divide your work into small, manageable sections.
Implement and test each section before moving on to the
next. A suggested order is as follows:
- Create the ColorGradientApp Class
- Implement a GradientPanel class that is simply a colored panel.
- Write the paintComponent method for Gradient panel that
draws a gradient for two default colors (red and white).
- Create a simple ColorButtonPanel that initializes 2 default colors.
- Add a single ColorButtonPanel object to your Gradient Panal and
verify
that you can change between colors
- Update the ColorButtonPanel to initialize 4+ default colors.
- Add the second ColorButtonPanel object to your Gradient Panel;
verify
that you can change between colors.
- Implement the Customize components on the ColorButtonPanel >
Grading As usual your program will be graded on both style and
correctness
Academic Integrity Reminder: Programming assignments are to
be
your own work. You may get help on the specifics of the assignment
from no one except the instructor. You may not show your program to
anyone or look at anyone's program or share ideas with anyone about how
to
write the program.
What to turn in
Turn in hardcopy of your .java files. Tar up your assign6
directory
and e-mail it to your instructor